icon_smt_section#
[ ]:
# Jupyter Notebook with widget matplotlib plots
#%matplotlib notebook
# Jupyter Lab with widget matplotlib plots
#%matplotlib widget
# with Jupyter and Jupyter Lab but without widget matplotlib plots
%matplotlib inline
%load_ext autoreload
%autoreload 2
[2]:
import sys
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from netCDF4 import Dataset, num2date
from ipdb import set_trace as mybreak
import pyicon as pyic
import cartopy.crs as ccrs
import glob
import pickle
import maps_icon_smt_temp as smt
import datetime
from icon_smt_levels import dzw, dzt, depthc, depthi
calc
tb
sys
scipy
matplotlib
cartopy
xarray
done loading
IconData
plotting
view
calc
tb
IconData
plotting
view
quickplots
quickplots
[ ]:
run = 'ngSMT_tke'
savefig = False
path_fig = '../pics/'
nnf=0
path_data = f'/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/????-??/'
path_ckdtree = ''
#fpath_tgrid = '/mnt/lustre01/work/mh0287/users/leonidas/icon/submeso/grid/cell_grid-OceanOnly_SubmesoNA_2500m_srtm30-icon.nc'
fpath_tgrid = '/home/mpim/m300602/work/icon/grids/smt/smt_tgrid.nc'
fpath_Tri = '/mnt/lustre01/work/mh0033/m300602/tmp/Tri.pkl'
[ ]:
lon_reg_1 = [-88, 0]
lat_reg_1 = [ 5, 68]
lon_reg_2 = [-75, -55]
lat_reg_2 = [30, 40]
lon_reg_3 = [-72, -68]
lat_reg_3 = [33, 35]
[ ]:
# --- load times and flist
search_str = f'{run}_T_S_sp_001-016_*.nc'
flist = np.array(glob.glob(path_data+search_str))
flist.sort()
times, flist_ts, its = pyic.get_timesteps(flist, time_mode='float2date')
[ ]:
if False:
# --- load tgrid
ts = pyic.timing(ts, 'load tgrid')
f = Dataset(fpath_tgrid, 'r')
clon = f.variables['clon'][:].astype(np.float32) * 180./np.pi
clat = f.variables['clat'][:].astype(np.float32) * 180./np.pi
clon_bnds = f.variables['clon_bnds'][:].astype(np.float32) * 180./np.pi
clat_bnds = f.variables['clat_bnds'][:].astype(np.float32) * 180./np.pi
f.close()
# --- cut tripolar grid
ts = pyic.timing(ts, 'cut to region')
ind_reg = np.where( (clon> lon_reg_3[0])
& (clon<=lon_reg_3[1])
& (clat> lat_reg_3[0])
& (clat<=lat_reg_3[1]) )[0]
#clon_reg = clon[ind_reg]
#clat_reg = clat[ind_reg]
clon_bnds_reg = clon_bnds[ind_reg,:]
clat_bnds_reg = clat_bnds[ind_reg,:]
# --- triangulation
ts = pyic.timing(ts, 'triangulation')
ntr = ind_reg.size
clon_bnds_rs = clon_bnds_reg.reshape(ntr*3)
clat_bnds_rs = clat_bnds_reg.reshape(ntr*3)
triangles = np.arange(ntr*3).reshape(ntr,3)
# --- save to temporary data
Tri = matplotlib.tri.Triangulation(clon_bnds_rs, clat_bnds_rs, triangles=triangles)
Tri.x = Tri.x.astype(np.float32)
Tri.y = Tri.y.astype(np.float32)
Tri.ind_reg = ind_reg
#np.savez(fpath_Tri, Tri=Tri, ind_reg=ind_reg)
fo = open(fpath_Tri, 'wb')
pickle.dump(Tri, fo)
fo.close()
else:
# --- load from temporary data
#ddnpz = np.load(fpath_Tri, allow_pickle=True)
#Tri = ddnpz['Tri']
#ind_reg = ddnpz['ind_reg']
fi = open(fpath_Tri, 'rb')
Tri = pickle.load(fi)
ind_reg = Tri.ind_reg
fi.close()
Specify section#
[ ]:
f = Dataset(fpath_tgrid, 'r')
#clon = f.variables['clon'][:].astype(np.float32) * 180./np.pi
#clat = f.variables['clat'][:].astype(np.float32) * 180./np.pi
clon = f.variables['clon'][:] * 180./np.pi
clat = f.variables['clat'][:] * 180./np.pi
f.close()
[ ]:
# --- cut tripolar data
lon_reg = [-72, -70]
lat_reg = [33, 34.5]
step = 20
ind_reg = np.where( (clon> lon_reg[0])
& (clon<=lon_reg[1])
& (clat> lat_reg[0])
& (clat<=lat_reg[1]) )[0]
clon = clon[ind_reg]
clat = clat[ind_reg]
[ ]:
# --- derive section ckdtree
p1 = -71.8, 34.2
p2 = -70.7, 33.5
npoints = 100
lon_sec, lat_sec, dist_sec = pyic.derive_section_points(p1, p2, npoints)
dckdtree_c, ickdtree_c = pyic.calc_ckdtree(lon_i=clon, lat_i=clat,
lon_o=lon_sec, lat_o=lat_sec,
n_nearest_neighbours=1,
)
Plot horizontal data#
[ ]:
fpath = flist_ts[0]
f = Dataset(fpath, 'r')
var = 'T016_sp'
temp = f.variables[var][its[step],:]
f.close()
[ ]:
temp_reg = temp[Tri.ind_reg]
[ ]:
# --- interpolate to rectgrid
fpath_ckdtree = '/mnt/lustre01/work/mh0033/m300602/proj_vmix/icon/icon_ckdtree/rectgrids/smt_res0.02_180W-180E_90S-90N.npz'
ddnpz = np.load(fpath_ckdtree)
lon_002deg = ddnpz['lon']
lat_002deg = ddnpz['lat']
temp_002deg = pyic.apply_ckdtree(temp, fpath_ckdtree, coordinates='clat clon', radius_of_influence=1.).reshape(lat_002deg.size, lon_002deg.size)
temp_002deg[temp_002deg==0.] = np.ma.masked
[ ]:
# --- cut domain 1
i1r1 = (lon_002deg<lon_reg_1[0]).sum()
i2r1 = (lon_002deg<lon_reg_1[1]).sum()
j1r1 = (lat_002deg<lat_reg_1[0]).sum()
j2r1 = (lat_002deg<lat_reg_1[1]).sum()
lon_002deg_r1 = lon_002deg[i1r1:i2r1]
lat_002deg_r1 = lat_002deg[j1r1:j2r1]
temp_002deg_r1= temp_002deg[j1r1:j2r1,i1r1:i2r1]
# --- cut domain 2
i1r2 = (lon_002deg<lon_reg_2[0]).sum()
i2r2 = (lon_002deg<lon_reg_2[1]).sum()
j1r2 = (lat_002deg<lat_reg_2[0]).sum()
j2r2 = (lat_002deg<lat_reg_2[1]).sum()
lon_002deg_r2 = lon_002deg[i1r2:i2r2]
lat_002deg_r2 = lat_002deg[j1r2:j2r2]
temp_002deg_r2= temp_002deg[j1r2:j2r2,i1r2:i2r2]
# --- cut domain 3
i1r3 = (lon_002deg<lon_reg_3[0]).sum()
i2r3 = (lon_002deg<lon_reg_3[1]).sum()
j1r3 = (lat_002deg<lat_reg_3[0]).sum()
j2r3 = (lat_002deg<lat_reg_3[1]).sum()
lon_002deg_r3 = lon_002deg[i1r3:i2r3]
lat_002deg_r3 = lat_002deg[j1r3:j2r3]
temp_002deg_r3= temp_002deg[j1r3:j2r3,i1r3:i2r3]
[ ]:
# ---
clim = [-2,30]
ccrs_proj = ccrs.PlateCarree()
dpi = 250
fig, hca, hcb = smt.make_axes(lon_reg_1, lat_reg_1, lon_reg_2, lat_reg_2, lon_reg_3, lat_reg_3, dpi)
ii=-1
ii+=1; ax=hca[ii]; cax=hcb[ii]
hm1 = pyic.shade(lon_002deg_r1, lat_002deg_r1, temp_002deg_r1, ax=ax, cax=cax, clim=clim,
transform=ccrs_proj, rasterized=False)
cax.set_ylabel('temperature [$^o$C]')
ht = ax.set_title(times[step])
#clim = [16,21]
clim = [18,21]
ii+=1; ax=hca[ii]; cax=hcb[ii]
hm2 = pyic.shade(lon_002deg_r2, lat_002deg_r2, temp_002deg_r2, ax=ax, cax=0, clim=clim,
transform=ccrs_proj, rasterized=False)
plt.colorbar(mappable=hm2[0], cax=cax, orientation='horizontal')
cax.set_xlabel('temperature [$^o$C]')
ii+=1; ax=hca[ii]; cax=hcb[ii]
#hm3 = pyic.shade(lon_002deg_r3, lat_002deg_r3, temp_002deg_r3, ax=ax, cax=cax, clim=clim,
# transform=ccrs_proj, rasterized=False)
hm3 = pyic.shade(Tri, temp_reg, ax=ax, cax=cax, clim=clim,
transform=ccrs_proj, rasterized=False)
for ax in hca:
ax.plot(lon_sec, lat_sec, color='k')
Section#
Load section data#
[16]:
ickd_min = ickdtree_c.min()
ickd_max = ickdtree_c.max()
ickdtree_c += - ickd_min
ind_reg += -ickd_min
[17]:
ind_reg.max()
[17]:
30447476
[27]:
told = datetime.datetime.now()
# --- load data
nz = 80
levs = np.arange(1,nz+1)
temp_sec = np.ma.zeros((levs.size, npoints))
flev = [
[ 1, 16],
[ 17, 32],
[ 33, 48],
[ 49, 64],
[ 65, 80],
]
nn = 0
temp_p = np.ma.zeros((levs.size))
told = datetime.datetime.now()
for kk, lev in enumerate(levs):
var = 'T%03d_sp'%lev
if lev > flev[nn][1]:
nn += 1
tnew = datetime.datetime.now(); tdiff=tnew-told; told=tnew
print(var, ": ", tdiff)
fpath = flist_ts[step]
fpath = fpath.replace('001-016', '%03d-%03d'%(flev[nn][0],flev[nn][1]))
print(fpath)
f = Dataset(fpath, 'r')
temp_sec[kk,:] = f.variables[var][its[step],ickd_min:ickd_max+1][ickdtree_c]
temp = f.variables[var][its[step],20]
f.close()
# if False:
# # --- cut to region
# data = temp[ind_reg]
# # --- interp to section
# datai = pyic.apply_ckdtree_base(data, ickdtree_c, dckdtree_c, radius_of_influence=1000e3)
# else:
# datai = temp[ickdtree_c]
# # --- store
# temp_sec[kk,:] = datai
T001_sp : 0:00:00.000182
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T002_sp : 0:00:02.117068
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T003_sp : 0:00:02.088527
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T004_sp : 0:00:02.100733
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T005_sp : 0:00:02.046471
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T006_sp : 0:00:02.047710
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T007_sp : 0:00:02.212586
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T008_sp : 0:00:02.223803
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T009_sp : 0:00:02.241945
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T010_sp : 0:00:02.205155
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T011_sp : 0:00:02.244584
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T012_sp : 0:00:02.227093
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T013_sp : 0:00:02.185716
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T014_sp : 0:00:02.310757
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T015_sp : 0:00:02.211652
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T016_sp : 0:00:02.161490
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_001-016_20100110T010000Z.nc
T017_sp : 0:00:02.197103
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T018_sp : 0:00:02.561941
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T019_sp : 0:00:02.222354
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T020_sp : 0:00:02.249630
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T021_sp : 0:00:02.240825
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T022_sp : 0:00:02.218505
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T023_sp : 0:00:02.173234
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T024_sp : 0:00:02.120860
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T025_sp : 0:00:02.148064
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T026_sp : 0:00:02.236659
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T027_sp : 0:00:02.152135
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T028_sp : 0:00:02.113954
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T029_sp : 0:00:02.090748
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T030_sp : 0:00:02.053017
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T031_sp : 0:00:02.090332
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T032_sp : 0:00:02.164567
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_017-032_20100110T010000Z.nc
T033_sp : 0:00:02.119350
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T034_sp : 0:00:02.567910
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T035_sp : 0:00:02.182450
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T036_sp : 0:00:02.221712
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T037_sp : 0:00:02.183372
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T038_sp : 0:00:02.190534
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T039_sp : 0:00:02.213528
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T040_sp : 0:00:02.142276
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T041_sp : 0:00:02.134383
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T042_sp : 0:00:02.106767
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T043_sp : 0:00:02.160571
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T044_sp : 0:00:02.172911
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T045_sp : 0:00:02.173766
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T046_sp : 0:00:02.121796
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T047_sp : 0:00:02.134522
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T048_sp : 0:00:02.102434
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_033-048_20100110T010000Z.nc
T049_sp : 0:00:02.129631
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T050_sp : 0:00:02.158126
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T051_sp : 0:00:02.150950
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T052_sp : 0:00:02.124302
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T053_sp : 0:00:02.215999
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T054_sp : 0:00:02.199180
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T055_sp : 0:00:02.134689
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T056_sp : 0:00:02.629492
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T057_sp : 0:00:02.159552
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T058_sp : 0:00:02.219721
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T059_sp : 0:00:02.146281
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T060_sp : 0:00:02.156909
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T061_sp : 0:00:02.136304
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T062_sp : 0:00:02.112015
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T063_sp : 0:00:02.136208
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T064_sp : 0:00:02.135957
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_049-064_20100110T010000Z.nc
T065_sp : 0:00:02.115578
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T066_sp : 0:00:02.113505
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T067_sp : 0:00:02.086113
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T068_sp : 0:00:02.072441
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T069_sp : 0:00:02.072196
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T070_sp : 0:00:02.056481
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T071_sp : 0:00:02.196113
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T072_sp : 0:00:02.097227
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T073_sp : 0:00:02.043157
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T074_sp : 0:00:02.068216
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T075_sp : 0:00:02.040751
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T076_sp : 0:00:02.057753
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T077_sp : 0:00:02.026119
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T078_sp : 0:00:02.013969
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T079_sp : 0:00:02.407204
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
T080_sp : 0:00:02.081833
/mnt/lustre01/work/mh0287/users/leonidas/icon/ngSMT/results/2010-01/ngSMT_tke_T_S_sp_065-080_20100110T010000Z.nc
[28]:
if False:
told = datetime.datetime.now()
fpath = flist_ts[step]
f = Dataset(fpath, 'r')
for kk in range(80):
#tnew = np.datetime64('now')
tnew = datetime.datetime.now(); tdiff=tnew-told; told=tnew
print(kk, ': ', tdiff)
temp = f.variables['T016_sp'][0,0]
f.close()
Plot section data#
[29]:
#idist = pyic.indfind(np.arange(0,120,40), dist/1e3)
idist = np.arange(npoints)[::40]
[30]:
hca, hcb = pyic.arrange_axes(1,1, plot_cb=True, asp=0.5, fig_size_fac=3)
ii = -1
# clim = 'auto'
clim = [14, 21]
ii+=1; ax=hca[ii]; cax=hcb[ii]
depth_sec = depthc[:levs.size]
hm = pyic.shade(dist_sec/1e3, depth_sec, temp_sec, ax=ax, cax=cax, clim=clim, contfs=np.linspace(clim[0],clim[1],21))
ax.set_ylim(depth_sec.max(),0)
ax.set_title('temperature $^o$C')
ax.set_xlabel('distance along section [km]')
ax.set_ylabel('depth [m]')
# cols = plt.cm.get_cmap('jet')(np.linspace(0,1,idist.size))
# for ii in range(idist.size):
# ax.axvline(dist_sec[idist[ii]]/1e3, color=cols[ii,:])
[30]:
Text(0, 0.5, 'depth [m]')
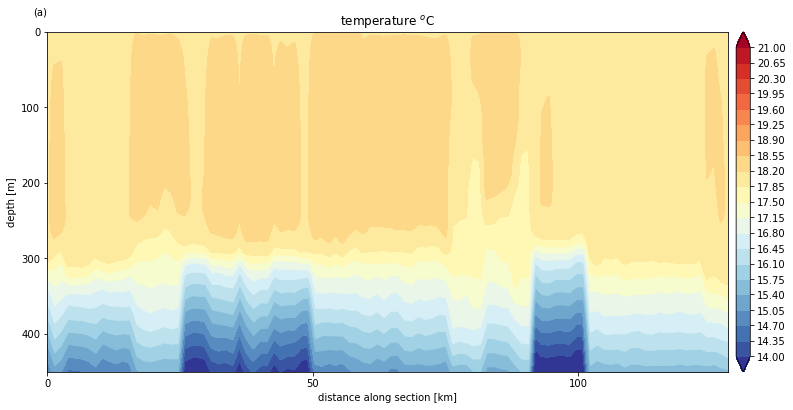
[25]:
temp_sec.max()
[25]:
0.0
Plot profiles#
[64]:
hca, hcb = pyic.arrange_axes(1,1, plot_cb=False, asp=1.2, fig_size_fac=3)
ii = -1
ii+=1; ax=hca[ii]; cax=hcb[ii]
for ii in range(idist.size):
ax.plot(temp_sec[:nz,idist[ii]], depthc[:nz], color=cols[ii,:])
#ax.set_ylim(depthc[:levs.size].max(), 0)
# ax.set_ylim(depthc[nz].max(), 0)
ax.set_ylim(300., 0)
ax.set_xlim(13,21)
ax.set_title('temperature [$^o$C]')
ax.set_ylabel('depth [m]')
[64]:
Text(0, 0.5, 'depth [m]')
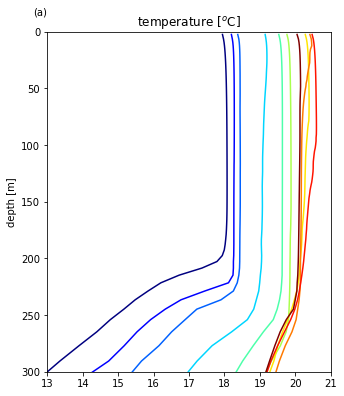
[34]:
temp_sec[:,idist[ii]].shape
[34]:
(80,)
[ ]: